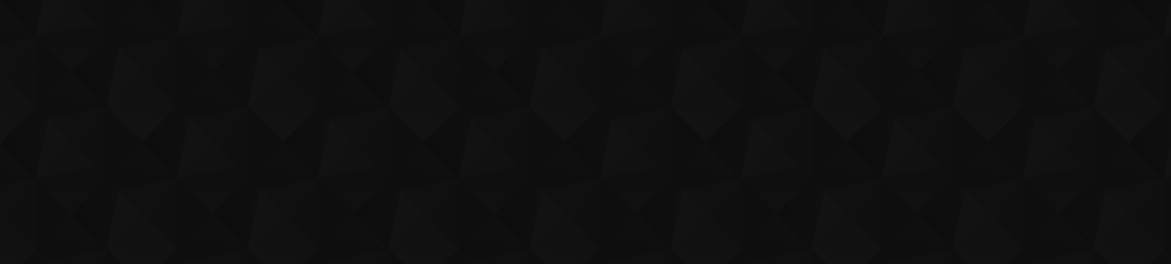
- 5
- 22 328
Code Flu
Romania
Registrace 15. 12. 2019
Quick Sort Algorithm in C#
In this video I explain how the Quick Sort algorithm works, in a visual way. At the end of the video I also provide the implementation in C#.
If you like this video, please consider taking my course on Data Structures using C# and .NET Core 3.0:
www.udemy.com/course/data-structures-using-csharp-and-dotnet/?referralCode=16E87C0830C3900103AA
If you like this video, please consider taking my course on Data Structures using C# and .NET Core 3.0:
www.udemy.com/course/data-structures-using-csharp-and-dotnet/?referralCode=16E87C0830C3900103AA
zhlédnutí: 8 025
Video
Merge Sort Algorithm in C#
zhlédnutí 7KPřed 4 lety
In this video I explain how the Merge Sort algorithm works, in a visual way. At the end of the video I also provide the implementation in C#. If you like this video, please consider taking my course on Data Structures using C# and .NET Core 3.0: www.udemy.com/course/data-structures-using-csharp-and-dotnet/?referralCode=16E87C0830C3900103AA
Insertion Sort Algorithm in C#
zhlédnutí 2,4KPřed 4 lety
In this video I explain how the Insertion Sort algorithm works, in a visual way. At the end of the video I also provide the implementation in C#. If you like this video, please consider taking my course on Data Structures using C# and .NET Core 3.0: www.udemy.com/course/data-structures-using-csharp-and-dotnet/?referralCode=16E87C0830C3900103AA
Selection Sort Algorithm in C#
zhlédnutí 1,4KPřed 4 lety
In this video I explain how the Selection Sort algorithm works, in a visual way. At the end of the video I also provide the implementation in C#. If you like this video, please consider taking my course on Data Structures using C# and .NET Core 3.0: www.udemy.com/course/data-structures-using-csharp-and-dotnet/?referralCode=16E87C0830C3900103AA
Bubble Sort Algorithm in C#
zhlédnutí 3,6KPřed 4 lety
In this video I explain how the Bubble Sort algorithm works, in a visual way. At the end of the video I also provide the implementation in C#. If you like this video, please consider taking my course on Data Structures using C# and .NET Core 3.0: www.udemy.com/course/data-structures-using-csharp-and-dotnet/?referralCode=16E87C0830C3900103AA
Finally a simple explenation with good visuals
public static void MergeSort(int[] array, int left, int right) { if(left < right) { int middle = (left + (right - 1)) / 2 + 1; MergeSort(array, left, middle - 1); MergeSort(array, middle, right); Merge(array, left, middle, right); } } public static void Merge(int[] array, int left, int middle, int right) { int leftIterator, rightIterator, arrayIterator; int leftArrayLength = middle - left; int rightArrayLength = right - middle + 1; int[] leftArray = new int[leftArrayLength]; int[] rightArray = new int[rightArrayLength]; for(leftIterator = 0; leftIterator < leftArrayLength; leftIterator++) { leftArray[leftIterator] = array[left + leftIterator]; } for(rightIterator = 0; rightIterator < rightArrayLength; rightIterator++) { rightArray[rightIterator] = array[middle + rightIterator]; } leftIterator = 0; rightIterator = 0; arrayIterator = left; while(leftIterator < leftArrayLength && rightIterator < rightArrayLength) { if (leftArray[leftIterator] <= rightArray[rightIterator]) { array[arrayIterator++] = leftArray[leftIterator++]; } else { array[arrayIterator++] = rightArray[rightIterator++]; } } int remainderIterator; if(leftIterator == leftArrayLength) { for(remainderIterator = rightIterator; remainderIterator < rightArrayLength; remainderIterator++) { array[arrayIterator++] = rightArray[remainderIterator]; } } if(rightIterator == rightArrayLength) { for (remainderIterator = leftIterator; remainderIterator < leftArrayLength; remainderIterator++) { array[arrayIterator++] = leftArray[remainderIterator]; } } }
What an explanation, great, the best, I was looking for quicksort for the past 2 days, now I have understood, thank you.
wow, you are the true algorithm king! thank you so much
Can You shard this wpf code for displaying the algorithme. I want to make the same but in blazor ? Thanks
I have test tmrw, thank you so so so much love you
Nice video well-done
What if i wanted it to arrange in discending order? please help)
In the partition function you need to replace if(a[i]<pivot) with if(a[i]>pivot)
@@codeflu2460 thank you very much. It worked. I had to change both the a[i]<pivot and a[i]>pivot.
How did you make visualization like this?
@@antonmartynenko8795 I wrote an app in WPF
I got StackOverFlow exception
The calculation for m is wrong , make it m = (l+(r-1))/2+1 *added the ()
Smart man
🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏🙏
Thank you for your explanation
Thanks a lot
amazing video
amazing video, I'm sorry you don't have more views on this...